The Ionic Framework is an open-source UI toolkit with integrations for popular frameworks such as Angular and React to build fast, high-quality applications using web technologies. Ionic allows for cross-platform development using either Cordova or Capacitor, with the latter supporting the development of desktop applications using Electron.
In this article, we will explore Ionic's integration with the React by creating an app that uses the Marvel Comics API to view comics and enable users to create a list of their favorites. We will also learn how to incorporate native capabilities with Capacitor into our software, and create builds for a native platform.
Requirements:
Before you can start using the Ionic Framework to create applications, you'll need the following:
1. Node.js built on your computer (at least v10)
2. Reacting Working Awareness
3. Staying familiar with the Hooks API
4. Some TypeScript Erfahrung
5. Native Android IDE, Android Studio, or XCode for iOS
6. An API-key Marvel Developer account.
Installing Ionic CLI
Ionic apps are primarily produced and built via the Ionic Command line Interface ( CLI). The CLI provides a wide variety of design tools and options for support as you build your hybrid application. You'll need to ensure that the CLI is enabled and accessible from your terminal to continue with this guide.
Open a new terminal window, and execute the following:
npm install -g @ionic/cli
This will update the new version of the Ionic CLI, and make it available on your computer from anywhere. If you want to confirm the success of the installation, the following command can be executed:
ionic –version
Starting An Ionic React Application:
Using the CLI it's easy to create an Ionic React application. It provides a command named start that creates files based on the JavaScript framework you choose for a new project. You may also choose to start with a pre-built UI prototype instead of the normal blank "Hello world" setting.
To proceed, conduct the following command:
ionic start marvel-client tabs --type=react –capacitor
Using the prototype tabs this command will create a new Ionic React device. It also adds an integration with Capacitor to your app. Capacitor is a cross-platform application runtime that makes it easy to run web apps native to iOS, Android and desktop.
Navigate to the newly generated directory on your terminal and start the server.
cd marvel-client
ionic serve
To see your app running, point your browser to http:/localhost:8100.
Note: If you used the create-react-app (CRA) before, you will feel very comfortable with the directory structure of your current project. That's because Ionic React projects are built using a setup similar to that found in a CRA device, to keep the creation experience familiar. React Router is also used under the hood for driving the navigation device.
Creating A React Component
In this step you will be building a reusable part of Respond. This part will receive the data and display comic details. This move is also intended to help show that Ionic React is only only Reacting.
Remove the files from src / components for the ExploreContainer portion and delete its imports from the.tsx files in the src / pages directory.
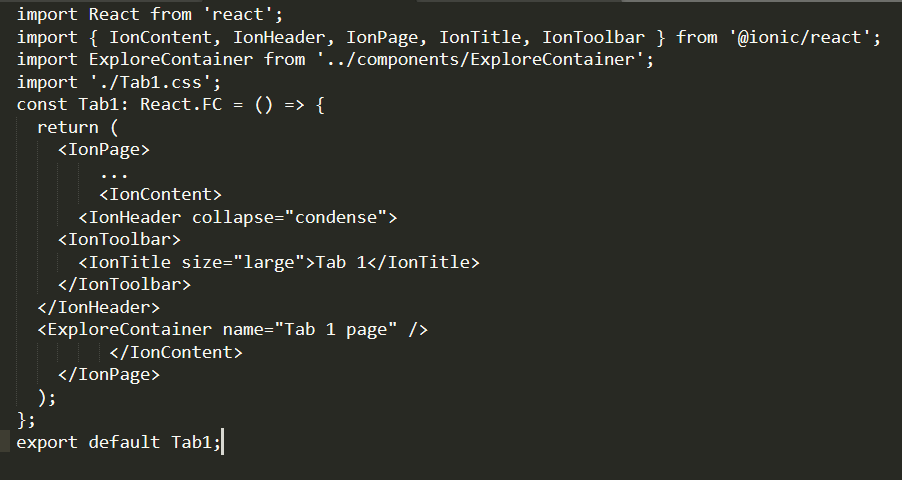
Only delete the content from the < IonContent></IonContent > tag in your Tab1.tsx format.
Next, build a file in your src / components directory called ComicCard.tsx. Then open the file in your editor and add the contents below:
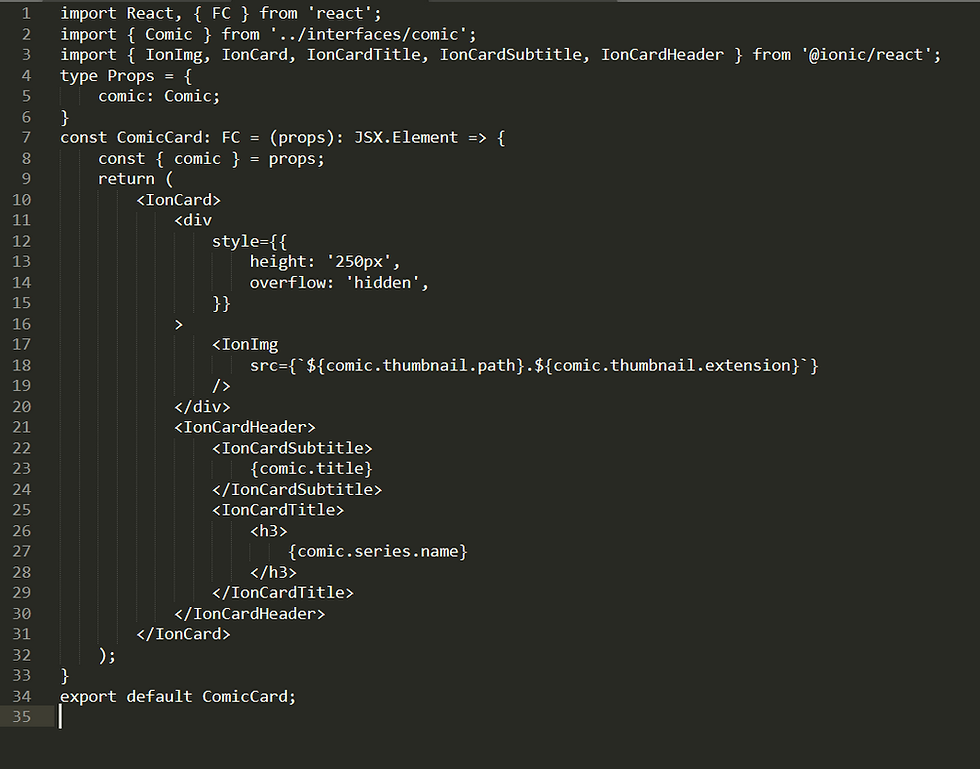
Your ComicCard component receives props containing comic details and uses an IonCard component to make the information. Cards are typically written in Ionic using certain subcomponents. In this code, you use the components IonCardTitle and IonCardSubtitle to render the comic title and series details inside a section of IonCardHeader.
Consuming The Marvel API
You will need to obtain some data from the Marvel API to use your newly developed feature. For the purposes of this tutorial, you can use the axios package to make your HTTP Requests. You will install it by running the command below:
yarn add axios
Next, add the following folder to your src directory:
# ~/Desktop/marvel-client/src
mkdir -p services
Then, cd into the services directory and create a file named api.ts:
# ~/Desktop/marvel-client/src/services
touch api.ts
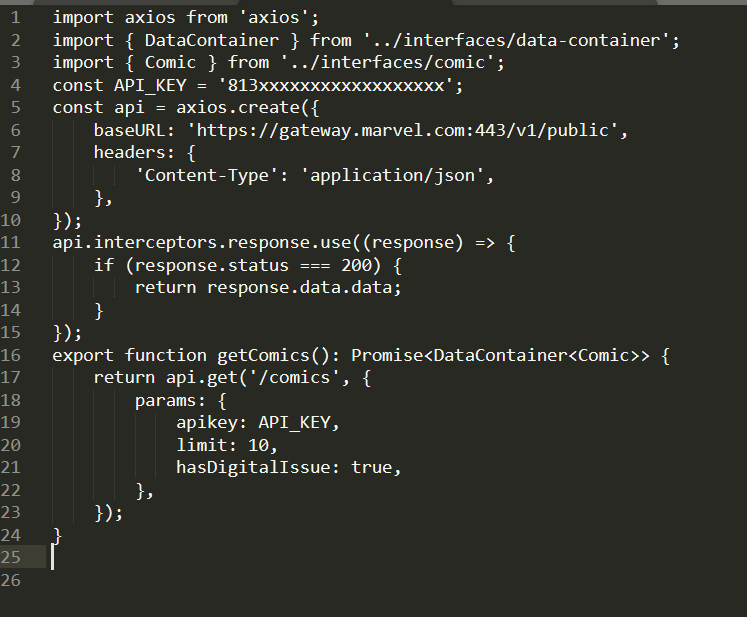
You should be sure to replace the API KEY value with your own API key. If you don't have one, you can apply by signing up on the website of the Marvel developers. You must also set up your account to allow requests from your local development server by adding localhost * to your approved Marvel referrers list.
You have now configured an axios instance to use the Marvel API. The api.ts file has only one export, reaching the GET / comics endpoint and returning a list of comics. You restrict outcomes to only those that are digitally visible. You can now continue with your transaction using the API Service.
Open the file Tab1.tsx and replace the contents by:
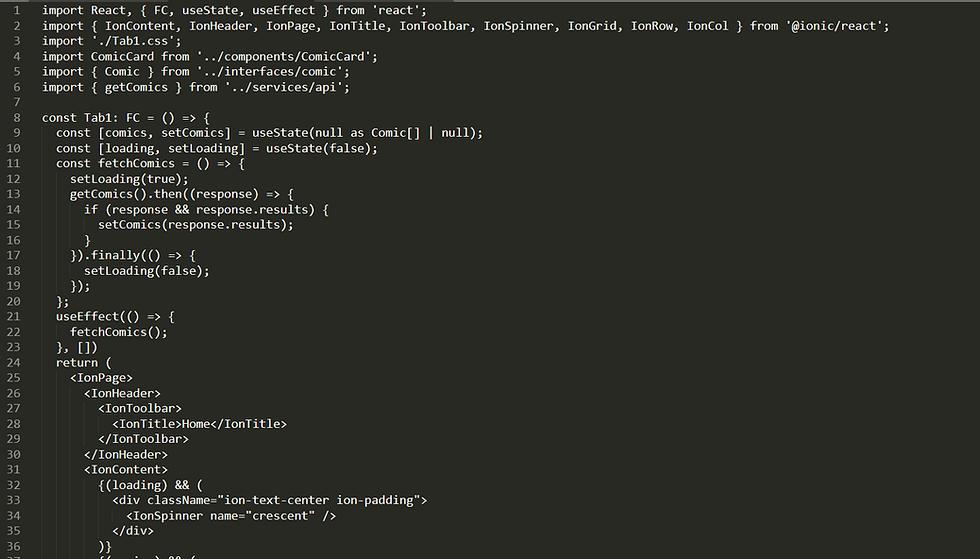

The above file is an example of an Ionic webpage. Pages are components accessible through a route / URL. To ensure the proper functioning of the transitions between pages, the IonPage component must be the root component of your page.
IonHeader is a part which should reside at the top of a page. Not needed for all pages, but it may contain useful components such as the page title, the link IonBackButton to move between pages, or the IonSearchBar. IonContent is the central field of content of your pages. It is responsible for providing the scrollable content in which users interact, plus any scrolling events that may be used in your app.
Within your part you have a function named fetchComics) (— called once within the useEffect) (hook — which allows a request by calling the getComics) (function you wrote earlier to get comics from the Marvel API. It saves the results through the usState) (hook to the state of your part. The part IonSpinner makes a spinning icon while your app asks the API to do so. When the request is completed, you pass the results on to the earlier generated ComicCard portion.
Build a small collection of fantastic comics
Your software so far looks decent but as a smartphone device it's not very useful. Within this move you are going to expand the functionality of your app by enabling users to 'star' comics, or save them as favorites. You can also use the Capacitor Storage plugin to make information about the saved favorites accessible to use offline.
First, create a file named util.ts in your src directory:
# ~/Desktop/marvel-client/src
touch util.ts
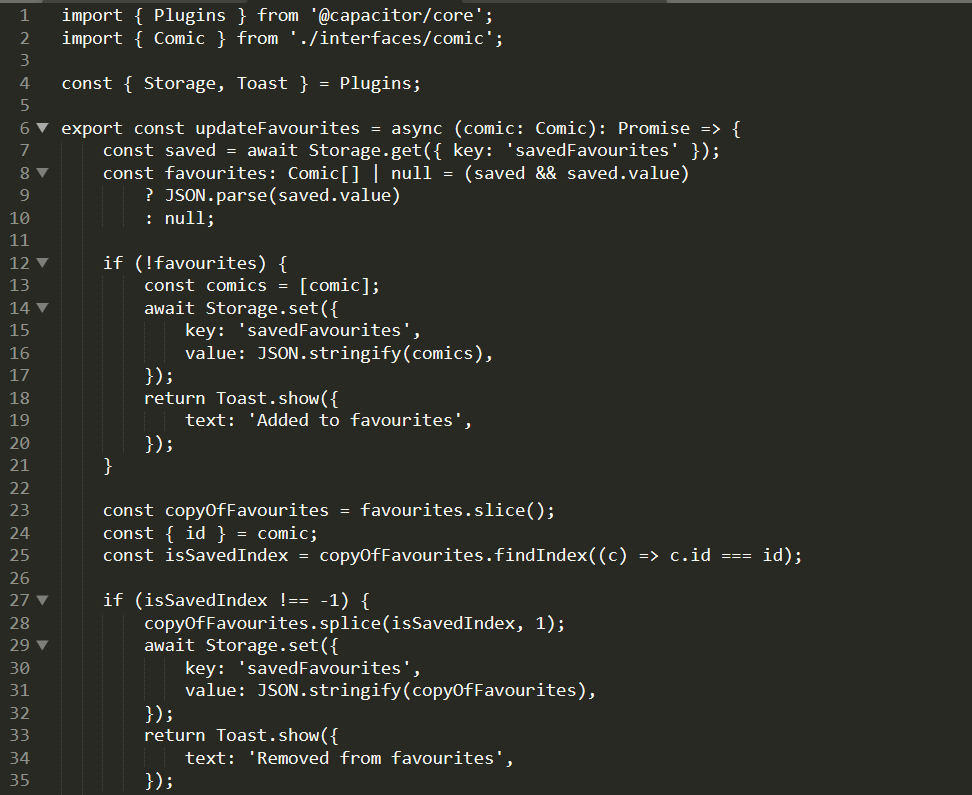
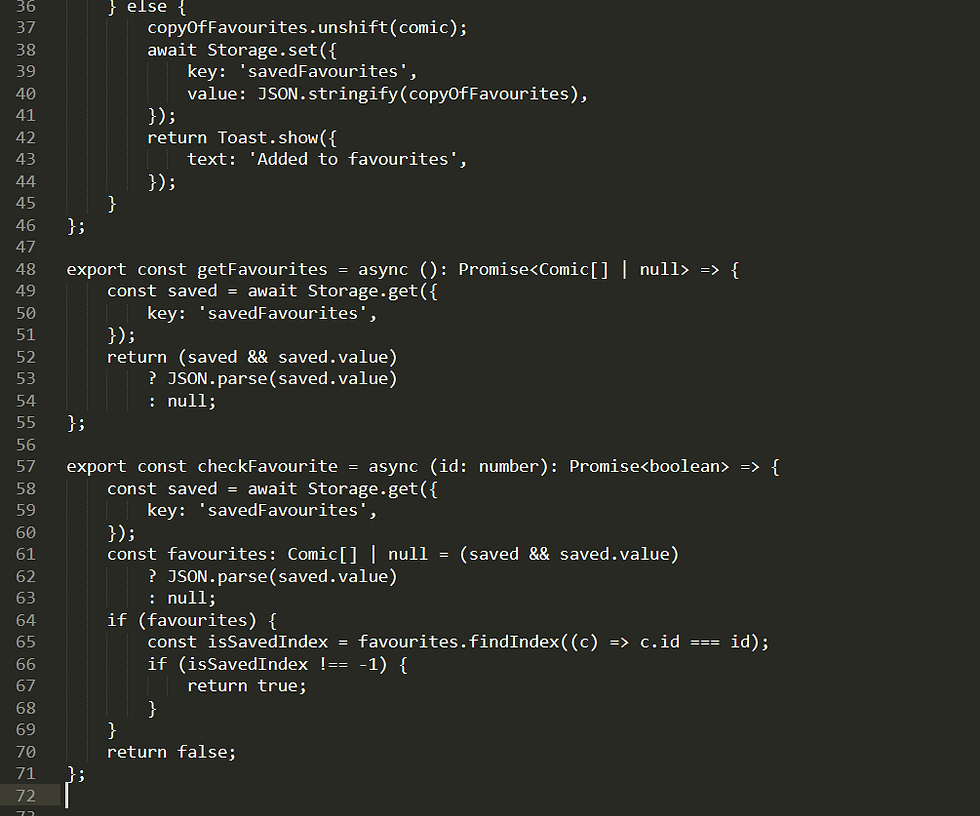
The Storage plugin provides a key-value store for basic data, while the Toast plugin provides a pop-up notification to show essential data to a user.
The updateFavourites) (function in this file takes a single argument, a comic object, and if it does not exist adds it to the device storage, or removes it from the system storage if it has already been saved. GetFavourites) (returns the saved comics of the user, while checkFavourites) (accepts a single argument, a Comic resource ID, and looks at it in the saved comics, returning true if it exists, or false if not.
First, open the ComicCard.tsx file and make the following modifications to enable users of your app to save their favorite comics:
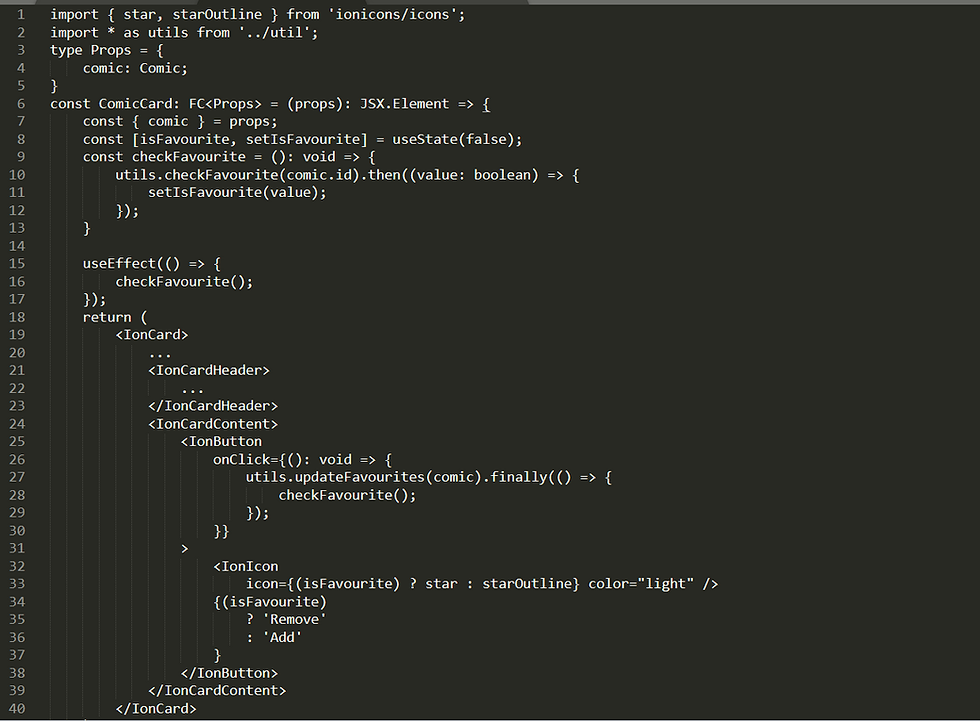
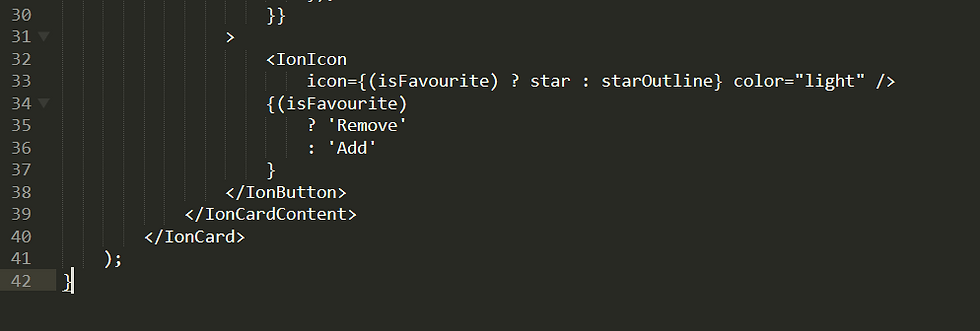
Your ComicCard component now has an IonButton feature that calls the updateFavourites) (function you wrote before when you clicked on it. Remember the feature works as a toggle, deleting the comic if it has already been saved, or else saving it. Do not forget to add the imports just added to this list for the new Ionic components IonButton, IonCardContent and IonIcon.
Now for the final part of this stage, where the saved comics will be made on their own page. Replace the contents of the file Tab2.tsx by:
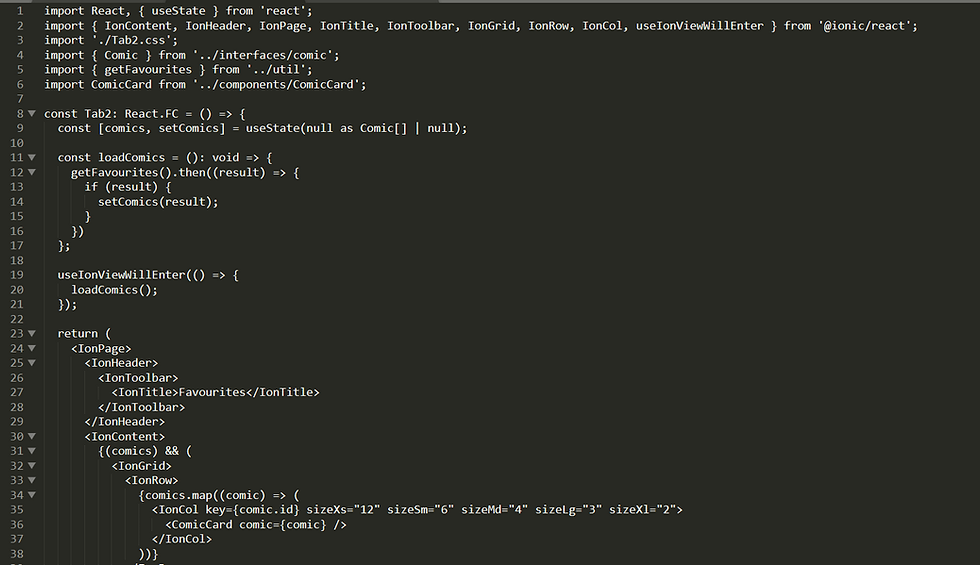
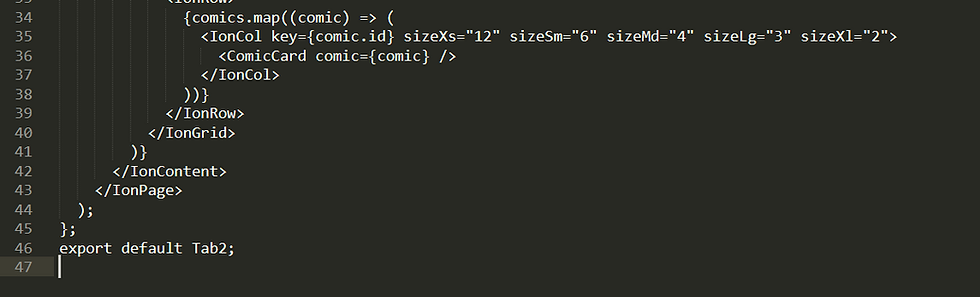
This page is very similar to the Tab1 page except you are accessing locally saved data instead of making an API request to get comics. You can use the Ionic life cycle loop, useIonViewWillEnter) (to make a call to the function that reads saved comics and updates the state of the variable instead of a useEffect) (switch. The hook to useIonViewWillEnter) (is called just as the page to be navigated enters view.
Your app now uses a few native plugins to boost its functionality. In the next step, you'll learn how to use Android Studio to generate a native project and create a native app.
Note: The * Tab3 * -based files can be deleted and the import and based * IonTab * portion removed in the * App.tsx * package.
Generating A Native Project
Ionic supports cross-platform device runtimes including Capacitor and Cordova. Such frameworks help you create and run on a native computer or emulator, apps built using Ionic. You will be using Capacitor to create native project files for the purposes of this tutorial.
You will need to produce a production construct of your application before you proceed with adding a server. To do so run the following command in the root directory of your project:
ionic build
Let's now add Capacitor to your project and generate the assets required to create a native application. Capacitor provides a CLI that can be accessed via npx or ionic CLI in your project as shown below:
USING ionic
Since you initialized your project using the --capacitor flag earlier, Capacitor has already been initialized with your project’s information. You can proceed to adding a platform by running the following command:
ionic capacitor add android
This command installs the dependencies necessary for android platform. This will also create the necessary files for a native Android project and copy the assets that you earlier developed while running ionic build.
If you have Android Studio installed, you can now open your Android Studio project, by running:
ionic capacitor open android
As a reputed Software Solutions Developer we have expertise in providing dedicated remote and outsourced technical resources for software services at very nominal cost. Besides experts in full stacks We also build web solutions, mobile apps and work on system integration, performance enhancement, cloud migrations and big data analytics. Don’t hesitate to get in touch with us!
This article is contributed by Ujjainee. She is currently pursuing a Bachelor of Technology in Computer Science . She likes most about Computer Engineering is that it allows her to learn and be creative. Also, She believes in sharing knowledge hence is very fond of writing technical contents for the same. Coding, analyzing and blogging are things which She can keep on doing!!
Comments