How to React to The HTML Drag-and - Drop API
- Whizzystack Solutions
- Jul 10, 2018
- 5 min read
One of the best features on HTML is the drag-and - drop API. It allows us to introduce drag-and - drop features in web browsers.
We are going to be dragging files from outside the browser in the current context. We put them on a list when dropping the file(s), and show their names. We could then perform some other procedure on the file(s) with the files in hand, e.g. upload them onto a cloud server.
In this tutorial, we will concentrate on how to incorporate the drag-and - drop action in a React program.
The dragenter, dragleave, dragover, And drop Events
The drag-and - drop events are eight different. That fires at another stage of the drag-and - drop process. In this tutorial, we will concentrate on the four that will be fired when an object falls into a drop zone: dragenter, dragleave, dragover and drop.
1. The dragenter event fires when a dragged item enters a valid drop target.
2. The dragleave event fires when a dragged item leaves a valid drop target.
3. The dragover event fires when a dragged item is being dragged over a valid drop target. (It fires every few hundred milliseconds.)
4. The drop event fires when an item drops on a valid drop target, i.e dragged over and released.
By defining the attributes for the ondragover and ondrop event handler, we can transform any HTML element into a valid drop target.
Drag-And-Drop Events In React
Check out branch 01-start. Verify that you do have yarn mounted. You can buy it at yarnpkg.com.
But if you prefer, create a new project React and replace the App.js material with the code below:

Replace App.css content with the CSS template below, too:
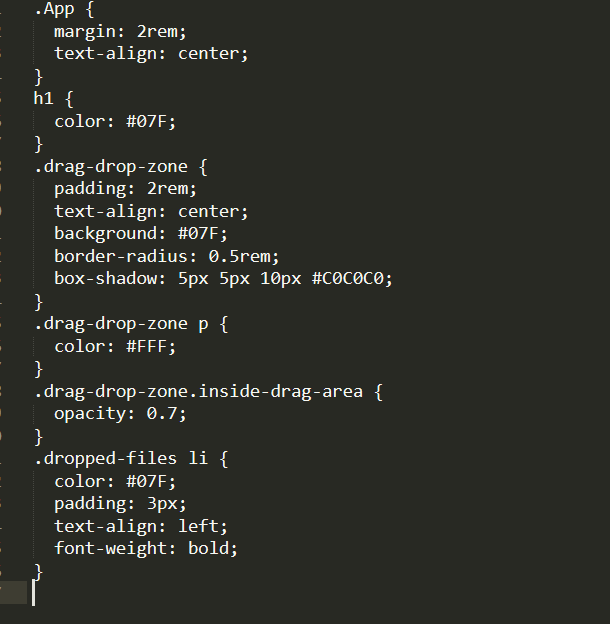
The next step is to build a part that will be drag and drop. Creates a DragAndDrop.js file inside the folder src/. Inside the file enter the following function:
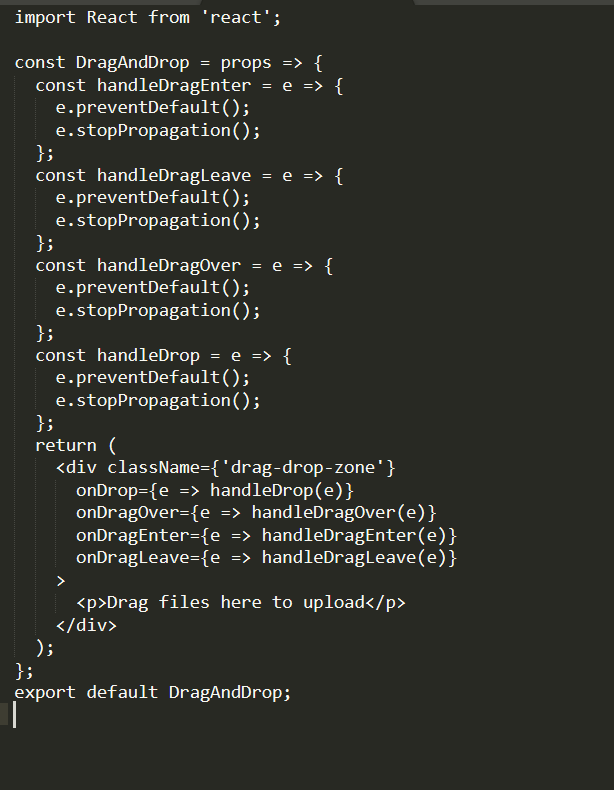
We have specified our HTML target event handler attributes in the return div. You can see, camel-casing is the only difference from pure HTML.
The div is now a legitimate drop goal as we have specified the attributes for the onDragOver and the event handler onDrop.
We also specified the functions for dealing with those events. Each of these handler functions receives the object as their argument for the case.
We call preventDefault) (for each of the event handlers to prevent the browser from executing its default behavior. The default behavior on the browser is to open the file fell. We also call stopPropagation) (to ensure the event does not spread from elements child to parent.
Import the DragAndDrop component into the App component and render it below the heading.
<div className="App">
<h1>React drag-and-drop component</h1>
<DragAndDrop />
</div>
Managing State With The useReducer Hook
Our next step is to write down the rationale for every one of our event handlers. Before we do that we need to remember how we plan to keep track of files that have been lost. This is where we start talking about the management of the Administration.
During the drag-and - drop process we must keep track of the following States:
1. dropDepth This will be an integer. We’ll use it to keep track of how many levels deep we are in the drop zone. Later on, I will explain this with an illustration
2. inDropZone This will be a boolean. We will use this to keep track of whether we’re inside the drop zone or not.
3. FileList This will be a list. We’ll use it to keep track of files that have been dropped into the drop zone.
React includes the usState and useReducer hooks for managing states. We are going to opt for the useReducer hook because we are going to deal with cases where a state depends on the prior one.
The useReducer hook accepts a form reducer (state, action) = > newState and returns the current condition coupled with a dispatch process.
Information on usingReducer can be read in the React docs.
Add the following code within the App portion (before returning a statement):
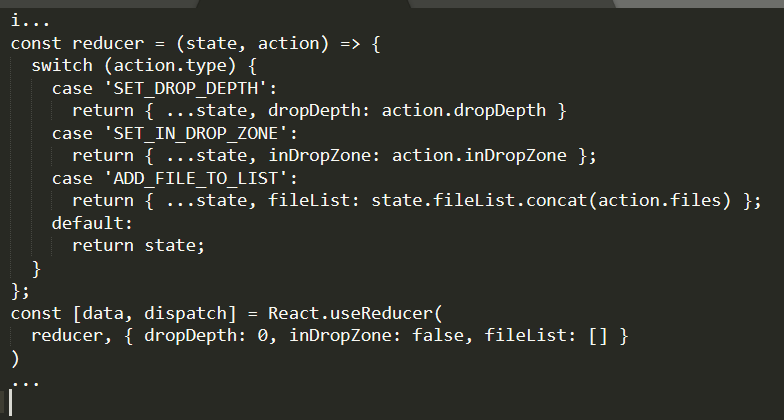
The useReducer setup accepts two arguments: an initial state and a reducer. It returns the current state and a dispatch feature to update the status. The state is updated by dispatching an action which contains an optional payload and a type. The change made to the state of the component is based upon what is returned as a result of the form of action from the case statement. (To remember that our initial condition is an object.)
To update it, we defined a corresponding case statement for each of the state variables. The update is performed by invoking the returned dispatch function usingReducer.
Now transfer data into the DragAndDrop feature you have in your App.js file and dispatch it as props:
<DragAndDrop data={data} dispatch={dispatch} />
At the top of the DragAndDrop component, we can access both values from props.
const { data, dispatch } = props;
If you’re following with the repo, the corresponding branch is 03-define-reducers.
Let’s finish up the logic of our event handlers. Note that the ellipsis represents the two lines:
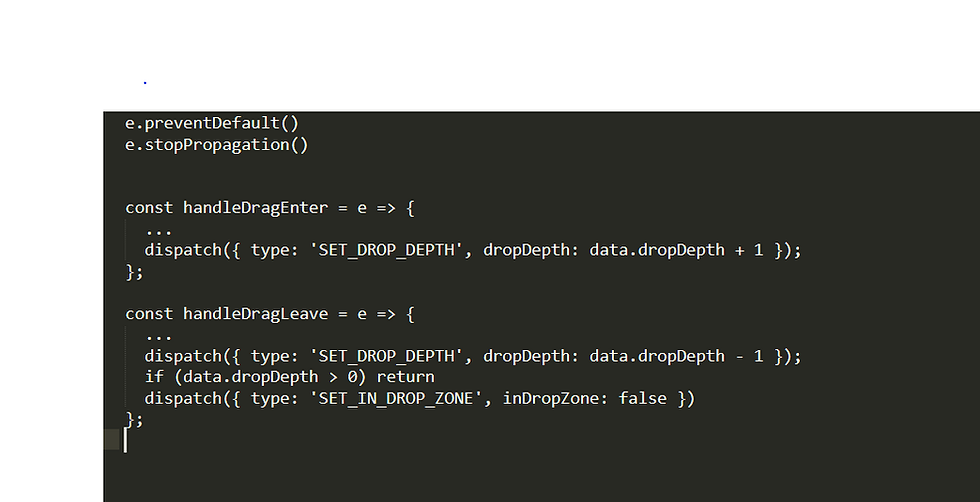
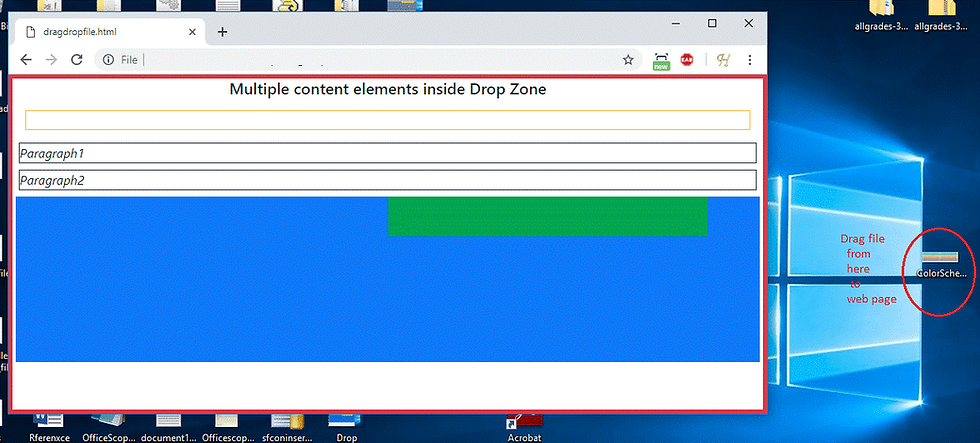
When dragging into a drop zone the ondragenter event is shot every time we reach a boundary. It occurs at both the A-in and B-in boundaries. When we reach the field, dropDepth is increased.
Likewise, when dragging out of a drop zone, the ondragleave event is fired each time we reach a boundary. This occurs at both the A-out and B-out boundaries. When we leave the field, we decrease dropDepth 's value. Note that at boundary B-out, we do not set inDropZone to false. Therefore we have this line to test the dropDepth and return from the dropDepth function greater than 0.
if (data.dropDepth > 0) return
This is because we are still inside zone A while the ondragleave event is set. It is only after we have reached A-out that we set inDropZone to false, and dropDepth is now 0. We have left all of the drop zones at this point.

We set inDropZone to true every time that this event fires. It tells us we are inside the drop line. We are also setting the dropEffect to be copied on the datatransfer file. It has the effect of having a green plus sign on a Mac, as you drag an object in the drop zone around.
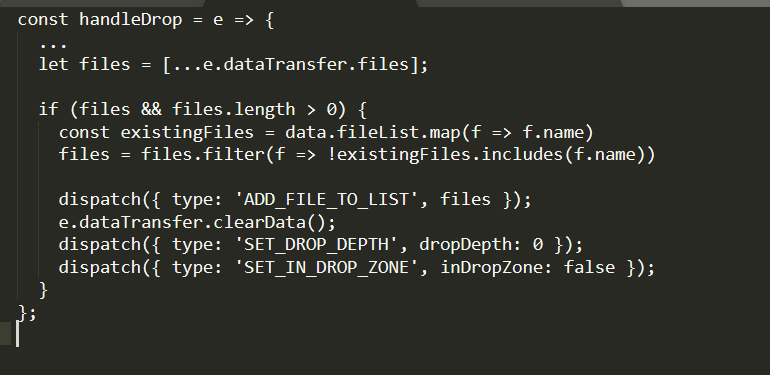
With e.dataTransfer.files we can access the files that are lost. The value is an array-like object, so that we convert it to a JavaScript array using the array spread syntax.
We will now test if there is at least one file before trying to add it to our file list. We also make sure that files which are already on our fileList are not included. The object DataTransfer is cleared for the next drag-and - drop operation. We reset the dropDepth and inDropZone values, as well.
Update div className in part DragAndDrop. It will modify div's className conditionally, depending on the data.inDropZone value.

Render the list of files in App.js by mapping through data.fileList.

Now try to drag and drop a few files into the drop line. When we reach the drop zone, you can see that the context is less opaque because the inside-drag-area class is allowed.
Concluding:
We've seen how the HTML drag-and - drop API is used to manage file uploads in React. We have also learned how to use the useReducer hook to control state. We may extend the handleDrop feature for the script. For eg, if we wanted to, we could add another check to restrict the file sizes. These can come before or after the current files test. Also, we could press the drop zone without affecting the drag-and - drop features.
As a reputed Software Solutions Developer we have expertise in providing dedicated remote and outsourced technical resources for software services at very nominal cost. Besides experts in full stacks We also build web solutions, mobile apps and work on system integration, performance enhancement, cloud migrations and big data analytics. Don’t hesitate to get in touch with us!
This article is contributed by Ujjainee. She is currently pursuing a Bachelor of Technology in Computer Science . She likes most about Computer Engineering is that it allows her to learn and be creative. Also, She believes in sharing knowledge hence is very fond of writing technical contents for the same. Coding, analyzing and blogging are things which She can keep on doing!!
Comments