Creating Sortable Tables With React
- Whizzystack Solutions
- Jul 10, 2018
- 4 min read
Sorting the table has always been a rather difficult problem to get right. To keep track of, detailed DOM mutations to do and even complex sorting algorithms, there are a lot of interactions. It is only one of those obstacles which is difficult to get correct. Exactly right?
Instead of pulling out into external libraries, let's try making things ourselves. We will be building a reusable way to sort your tabular data in React in this post. We will go through every move in detail, and learn along the way a bunch of useful techniques.
We're not going to go through basic React or JavaScript syntax, but to follow along, you don't have to be an expert in reacting.
Creating A Table With React
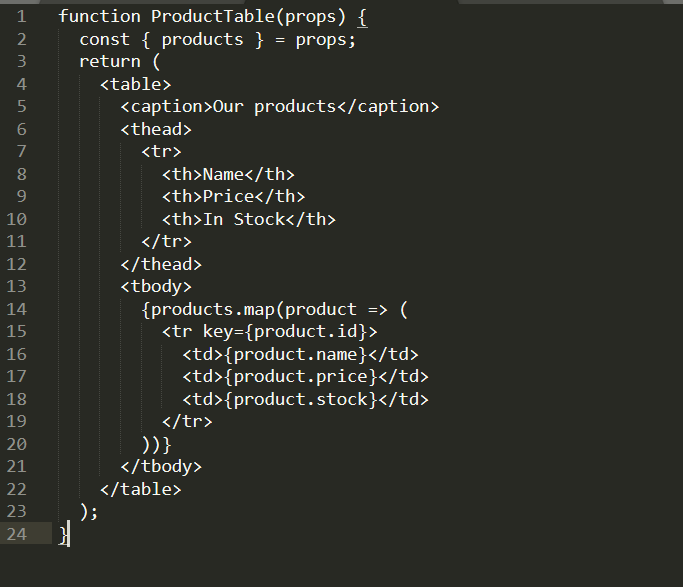
Sorting The Data
If you would believe all the interviewers on the whiteboard, you would think that software engineering has been almost all sorting algorithms. Fortunately, we're not going to look into a short type or type of bubble here.
Data sorting in JavaScript is quite straightforward, thanks to the sort) (function of the built-in array. Without an extra argument it will sort arrays of numbers and strings:
const array = ['mozzarella', 'gouda', 'cheddar'];
array.sort();
console.log(array); // ['cheddar', 'gouda', 'mozzarella']
If you want something a bit cleverer, a sorting function may be passed. This function is given as arguments to two things in the list, and positions one in front of the other based on what you decide.
Let's start by sorting out the data by name which we get alphabetically.
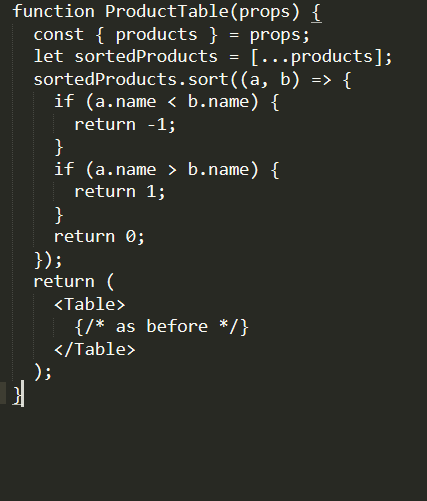
What's happening here, then? First, we create a copy of the prop products, which we can change and modify as we please. We need to do so because the method Array.prototype.sort replaces the original list, rather than returning a new sorted copy.
Making Our Table Sortable
So now we can make sure that the table is sorted by name, but how can we alter the sorting order ourselves?
We need to recall the field currently sorted to adjust by which field we sort by. We are going to do that with the useState fork.
A hook is a special form of feature that enables one to "hook" into some of the key features of React, such as state control and triggering side effects. This specific hook allows us to retain, and alter, a piece of internal state in our component if we like. That is what we will be adding:
const [sortedField, setSortedField] = React.useState(null);
We start by doing no sorting at all. Next, let's modify the table headings to include a way of changing by which field we want to sort.
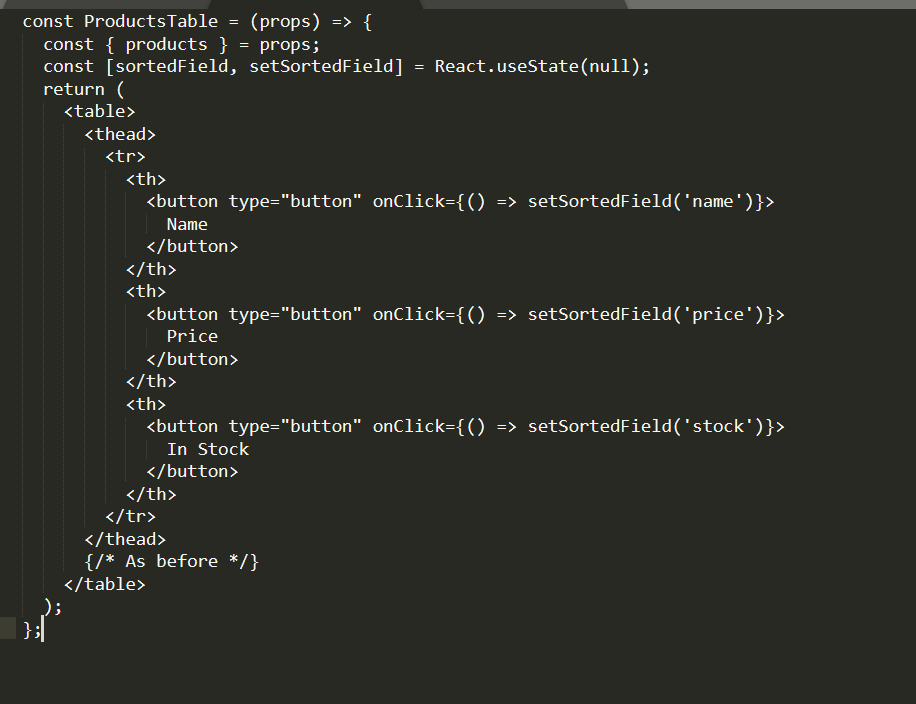
Now, whenever we click the heading of a table, we update the field we want to sort by. Neat-o-o!
However, we are still not doing any real sorting, so let's resolve that. Mind the algorithm of previous sorting? Here it is, altered only slightly to fit with some of our field names.
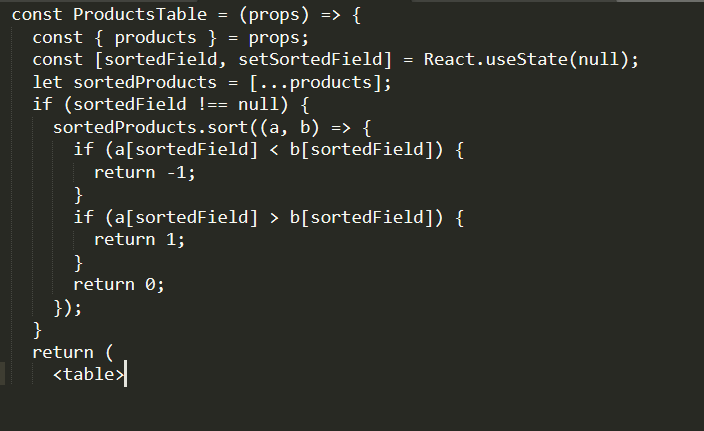
Ascending Vs Descending
The next feature we would like to see is a way to switch from ascending to descending order. By clicking the table heading one more time, we will switch between ascending and descending order.
We'll need to add a second piece of state to execute this — the sort order. To retain both the name of the field and its direction we must refactor our current sortedField state variable. This state variable will contain an object with a key (the field name) and a path, rather than a string. To be a little simpler we will rename it to sortConfig.
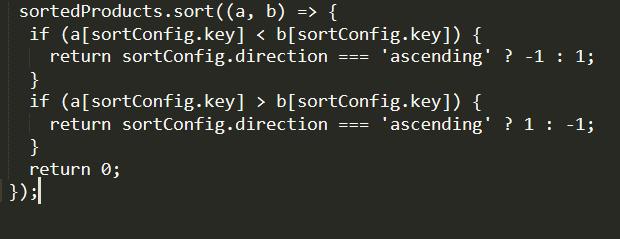
Now, if the course is 'ascending' we're going to do as we have done before. If it is not, we are going to do the reverse, giving us a downward direction.
Next we will create a new function — requestSort — that will accept the name of the field, and update the state accordingly.
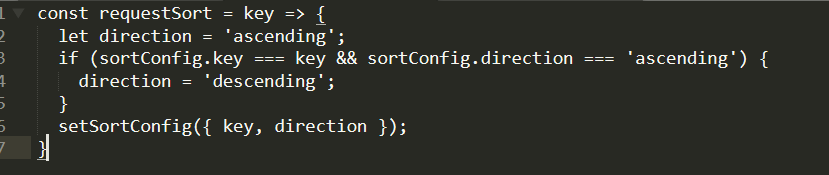
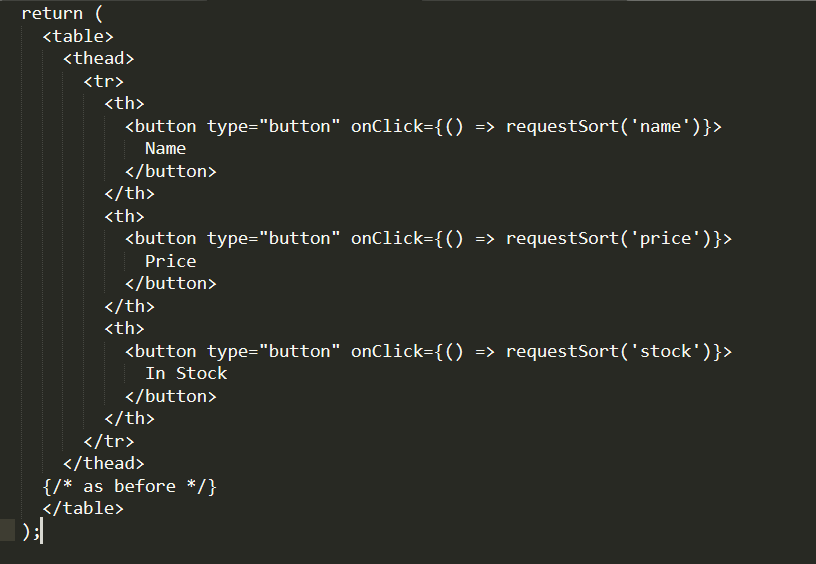
Now we are starting to look pretty feature-complete, but one big thing still remains to be done. We need to ensure we sort our data only when we need it. We are currently sorting out all our data on every render, which will lead to all kinds of performance problems down the line. Let's use the built in Memo hook instead to memorize all the slow bits!
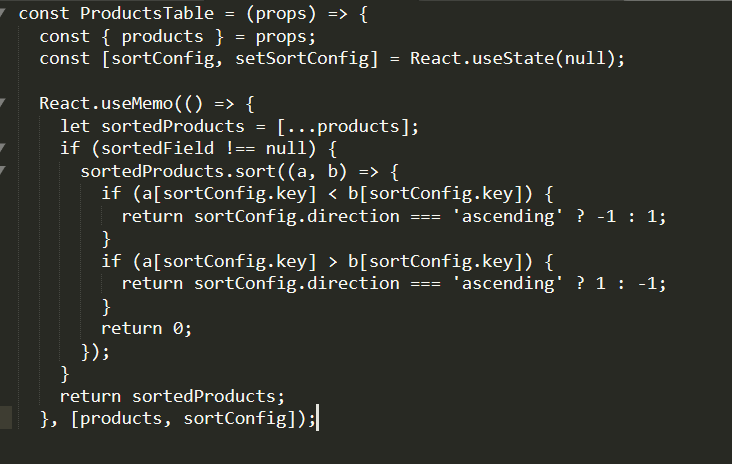
If you haven't seen it before, useMemo is a way to archive costly computations — or memoize them. So given the same data, if we re-render our component for some purpose it doesn't have to sort the products twice. Notice that if our goods change we want to cause a new type, or the field or path we sort by changes.
Wrapping our code in this feature will have major consequences for the efficiency of our table sorting!
Making It All Reusable
One of the best things about hooks is how simple it is to make reusable reasoning. You 're likely to be juggling all types of tables in your submission, and having to re-implement the same stuff sounds like a drag again.
React has the functionality called custom hooks. They sound fancy but they're all standard features that use other hooks inside. Let's refactor our code to include in a custom switch, so that we can use it anywhere!
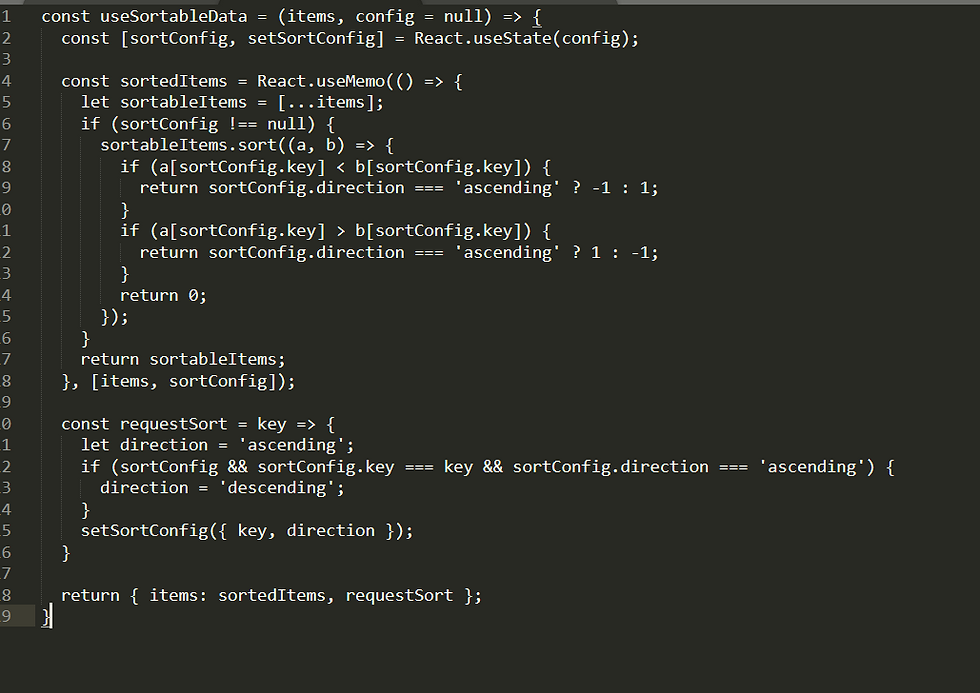
This is pretty much copy and paste from our previous code, with a bit of renaming thrown in. useSortableData accepts the items, and an optional initial sort state. It returns an object with the sorted items, and a function to re-sort the items.
Our table code now looks like this:
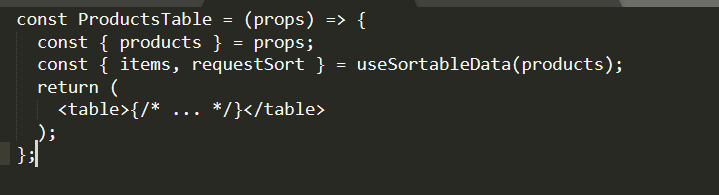
A Last Touch
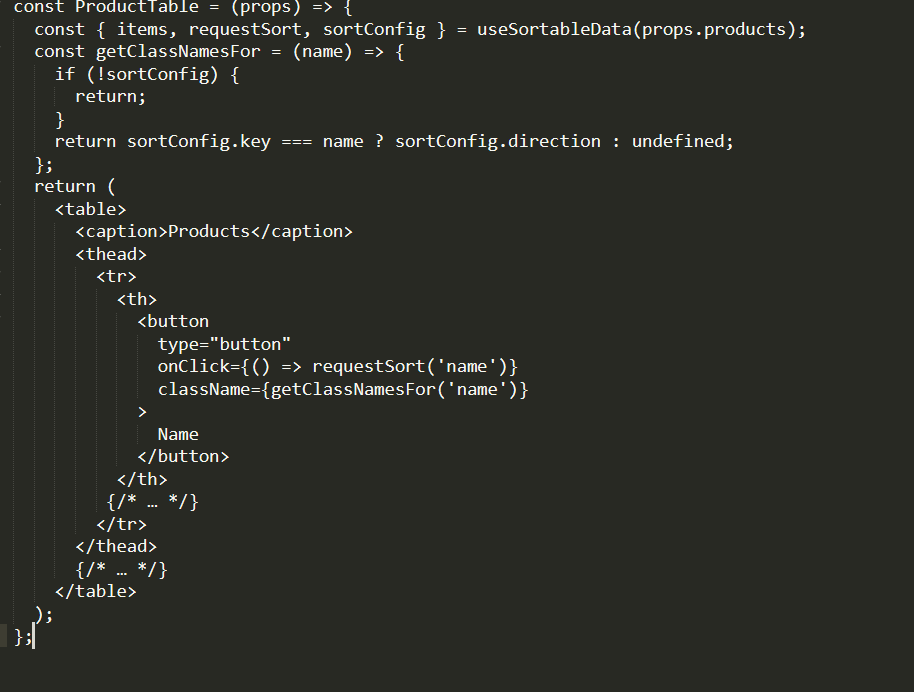
Wrapping Up
As it turns out, after all, building your own table sorting algorithm was not an unthinkable task. We found a way to model our state, we wrote a generic sorting function and we wrote a way of updating what our preferences for sorting are. We ensured everything was performing and refactured everything into a custom hook. Finally, we've provided the user with a way to show the sort order.
As a reputed Software Solutions Developer we have expertise in providing dedicated remote and outsourced technical resources for software services at very nominal cost. Besides experts in full stacks We also build web solutions, mobile apps and work on system integration, performance enhancement, cloud migrations and big data analytics. Don’t hesitate to get in touch with us!
This article is contributed by Ujjainee. She is currently pursuing a Bachelor of Technology in Computer Science . She likes most about Computer Engineering is that it allows her to learn and be creative. Also, She believes in sharing knowledge hence is very fond of writing technical contents for the same. Coding, analyzing and blogging are things which She can keep on doing!!
Comments